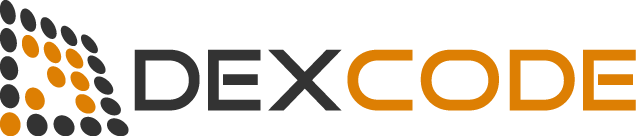
Decryptable password with Devise
When using Devise to handle user authentication for a Ruby on Rails application, Devise will transform password into a hash before stores it in database. The hashing process or encryption inside Devise, by default, is using BCrypt. BCrypt is a one-way hash function, means hashed password can not be decrypted and only user knows what the password is.
But, what if we want to know what the actual user password is?
We need to change the encryption function used by Devise and fortunately, Devise is flexible when it comes to encryptor options. One of the encryption that enable hash to be decrypted is aes256. Here is how to setup devise to use aes256 encryption:
Include required gems
# Gemfile
gem 'devise-encryptable'
gem 'aes', '~> 0.5.0'
Enable devise encryptable
In user model, add :encryptable
to devise:
# user.rb
devise :database_authenticatable, :registerable,
:recoverable, :rememberable, :trackable, :validatable,
:encryptable
Create new devise encryptor Aes256
# config/initializers/aes256.rb
require 'aes'
module Devise
module Encryptable
module Encryptors
class Aes256 < Base
class << self
def digest(password, stretches, salt, pepper)
::AES.encrypt(password, pepper, {:iv => salt})
end
alias :encrypt :digest
def salt(stretches)
::AES.iv(:base_64)
end
def decrypt(encrypted_password, pepper)
::AES.decrypt(encrypted_password, pepper)
end
end
end
end
end
end
Tell devise to use Aes256
as encryptor
# config/initializers/devise.rb
config.encryptor = :aes256
Setup done!
And then to decrypt a user password, create a method inside user class:
# user.rb
def decrypted_password
Devise::Encryptable::Encryptors::Aes256.decrypt(encrypted_password, Devise.pepper)
end
Dexcode is a Ruby on Rails outsourcing company. If you need help with your project whether it's maintaining existing project, creating MVP from scratch, writing unit tests and integration tests, reviewing existing codes and improving security, reliability & performance, don’t hesitate to contact us.